Published
- 2 min read
TypeScript2
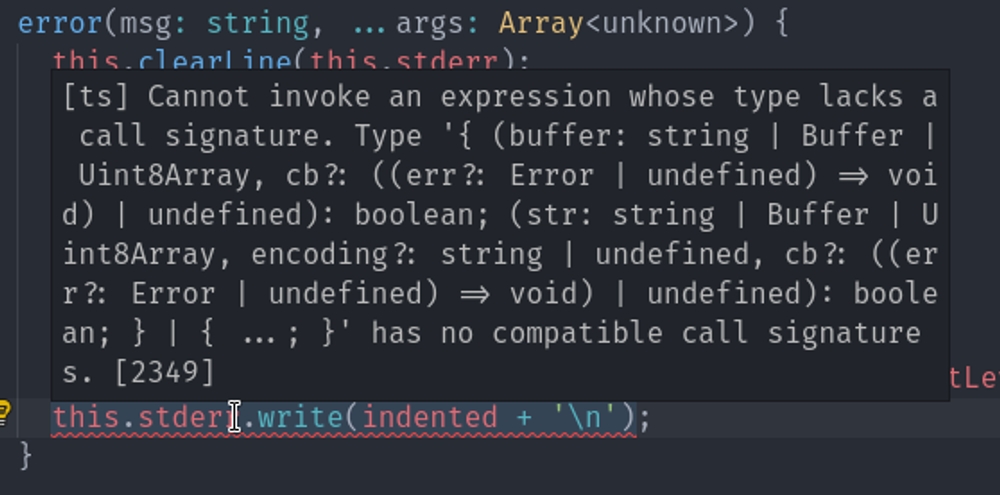
TypeScript: JavaScript with Superpowers
TypeScript is a programming language that builds on top of JavaScript, adding a powerful feature: types. It’s designed to improve code quality, maintainability, and developer productivity by catching potential errors early on during development.
Key Features:
- Static Typing: You explicitly declare the types of variables and function parameters, ensuring code consistency and preventing runtime errors.
- Type Inference: TypeScript often infers types automatically, saving you time and effort.
- Classes and Interfaces: Organize code with robust object-oriented constructs.
- Type Annotations: Add descriptive information to improve code readability and maintainability.
- Type Checking: Compiler flags potential type mismatches and errors before runtime.
- Transpiles to JavaScript: TypeScript code converts to plain JavaScript, running seamlessly in any JavaScript environment.
Code Examples:
1. Variable Types:
TypeScript
let greeting: string = “Hello, TypeScript!”; let age: number = 30; let isAdmin: boolean = true;
2. Function Types:
function add(x: number, y: number): number {
return x + y;
}
3. Classes and Interfaces:
TypeScript
interface Person {
name: string;
age: number;
}
class Employee implements Person
{
name: string;
age: number;
jobTitle: string;
constructor(name: string, age: number, jobTitle: string) {
this.name = name;
this.age = age;
this.jobTitle = jobTitle;
}
}
4. Type Annotations:
const myArray: number[] = [1, 2, 3];
const myObj: { name: string, age: number } = { name: "Alice", age: 25 };
Benefits of TypeScript:
- Improved Code Quality: Reduced errors and bugs, leading to more reliable applications.
- Better Tooling: Enhanced code completion, refactoring, and navigation in editors and IDEs.
- Improved Maintainability: Clearer code structure and type information for easier understanding and modification.
- Large-Scale Application Development: Particularly well-suited for complex projects with multiple developers.
Visual Representation of Type Checking:
Exploring TypeScript Further:
- Official TypeScript Website: https://www.typescriptlang.org/
- TypeScript Playground: https://www.typescriptlang.org/play
- TypeScript Tutorials: https://www.typescriptlang.org/docs/handbook/typescript-in-5-minutes.html
Sources
1. https://github.com/1bella2bella3bella/FE21-Sayuri-Isabella
2. https://github.com/FIT2095/content
Generated by Bard with propmt: can you tell me what typescript is with code examples
editRegenerate draftrefreshvolume_up